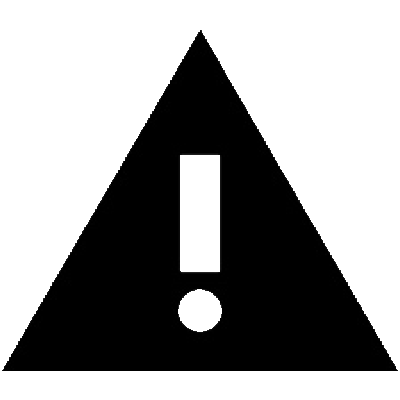
You must sign in via Discord to make contributions.
You cannot write code, conduct tests or make comments when logged out.
SIGN ININSTRUCTIONS
In this module, you will beta test some programs outside of this webpage. These programs are the main functionalities of the project. All you have to do is test them, look at them or play around with it! Everything is expected to work but the project compiler - This page will be updated every now and then to include new and updated components of the system for the project to run.
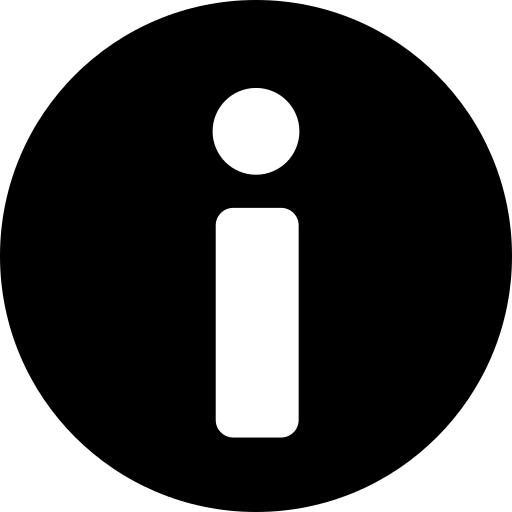
You can view all of these experiments on GitHub!
All source code is available for anyone to view.
TAKE A LOOKINSTRUCTIONS
In this module, you will see a screen that will preview demos. Your job is to test these demos to see if they work with your device. If a demo doesn't work, make a comment about it and be sure to share your device model!
These demos will have various device input controls involving:
• Touch / Mouse Testing - Tapping, dragging, clicking, etc.
• Controller Testing - Joystick movements, button presses, etc.
• Keyboard Testing - Keydown, key release, key hold, etc.
In addition, the preview screen will attempt to resize to the closest supported aspect ratio and fullscreen mode would be available.
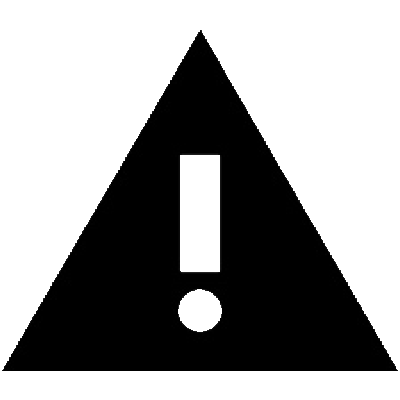
No demo loaded! Nothing to see here...
Please open a demo to preview.
DATA.UPVOTECOUNT
DATA.USERNAME
DATA.CREATIONDATE
DATA.COMMENT
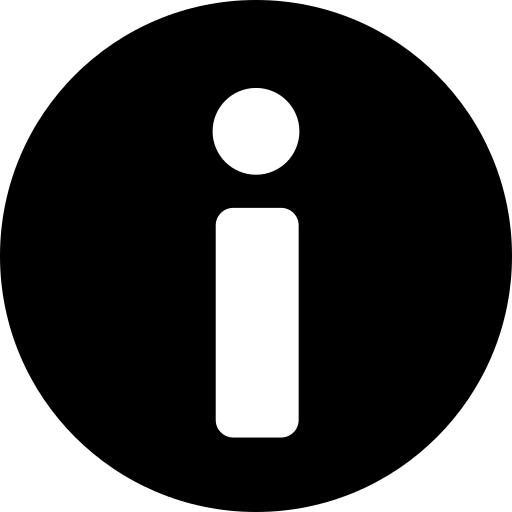
This Behavior has Hidden / Optional Inputs
Yellow input fields are hidden in most behaviors and may serve no functionality. Learn More
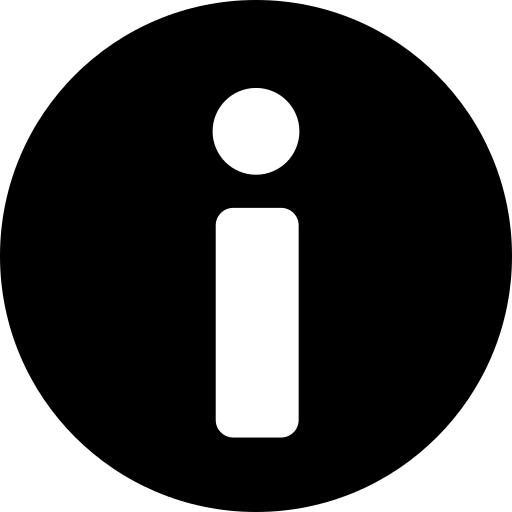
This Behavior has Hidden / Optional Inputs
Yellow input fields are hidden in most behaviors and may serve no functionality.
In hyperPad, some of these input fields are hidden from the user - almost never to be seen in the editor.
You may ignore these input fields as they provide very specific functionality to behaviors.
outputs: An array containing behavior IDs of behaviors using output(s) of this behavior.
storage: Additional meta data stored by the behavior. (Unsure)
values: A dictionary used to store outputs from other behaviors. Known to be used by Box Container.
symbol: A character used to show the operator between two operands. Known to be used by math behaviors like Add Values.
INPUT FIELDS
OUTPUT FIELDS
Loading Content
Comments (0)
SHOW SUBMISSIONS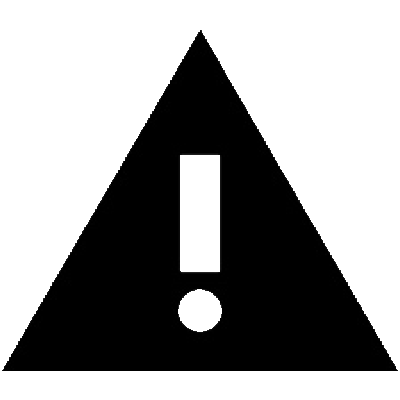
Hmmm...
Seems, like no one has commented on this behavior yet - be the first one!
INSTRUCTIONS
In this module, you can select a behavior to program - the way you program a behavior will determine its functionality. Once you select a behavior, you will be taken a page where you can see comments and code submissions from other users. At the top of the page, select Program Behavior to open it in a code editor. Documentation on how to use the behavior system will be in the Documentations tab - you may access it anytime.
In the code editor, you can write and run your code. To submit it, your code must follow certain criteria:
• Code cannot throw errors or have invalid syntax.
• Code must use some input fields - if any. Some input fields can be useless.
• Code must use all output fields - if any.
• Code cannot contain malware, bloatware or irrelevant functions.
STATUS KEYS
Each behavior on this page has a status indicated by a small colored circle.
•
No Progress: No one has started working on the behavior yet.
•
In-Progress: Users have submitted code that is awaiting verification.
•
Verified: A code snippet has been verified and will be implemented soon.
•
Implemented: The behavior has been 100% implemented in the latest version.
•
Cancelled: The behavior cannot be replicated on the web due to limitations.
•
Deprecated: Behavior is outdated and does not work in the latest version.
INTERACTION
0 Behaviors in this Category
Touch Input, Drag & Drop, Joystick, etc.
OBJECT
0 Behaviors in this Category
Tags, Object Collisions, Attributes, etc.
SCREEN
0 Behaviors in this Category
Screen Position, Rotation, Zoom, etc.
TRANSFORM
0 Behaviors in this Category
Object Position, Scale, Rotation, etc.
UI
0 Behaviors in this Category
Text, Popups, Life Indicators, Health Bars, etc.
FX
0 Behaviors in this Category
Particle FX, Sound FX, Animation, etc.
Scene
0 Behaviors in this Category
Scenes, Overlay, Time Scale, etc.
Physics
0 Behaviors in this Category
Physical Properties, Attachments, Modes, etc.
Logic
0 Behaviors in this Category
Math, Loops, Conditionals, etc.
Custom
0 Behaviors in this Category
Networking, Storage, Timers, etc.
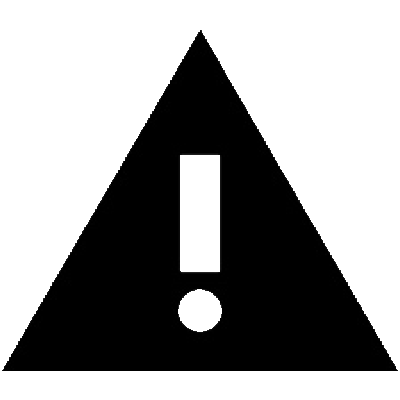
No behavior selected!
Please select a behavior to open in the IDE.
Active
Root
Global
Children Count
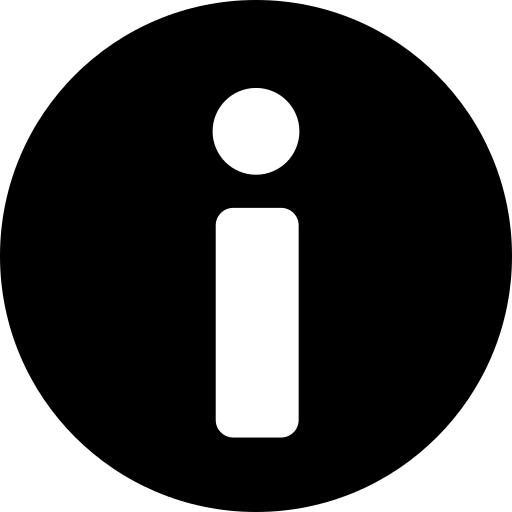
Good Thing to Note
The input and output fields below will highlight green if they are being utilized in the code. All output fields should be used in the code.
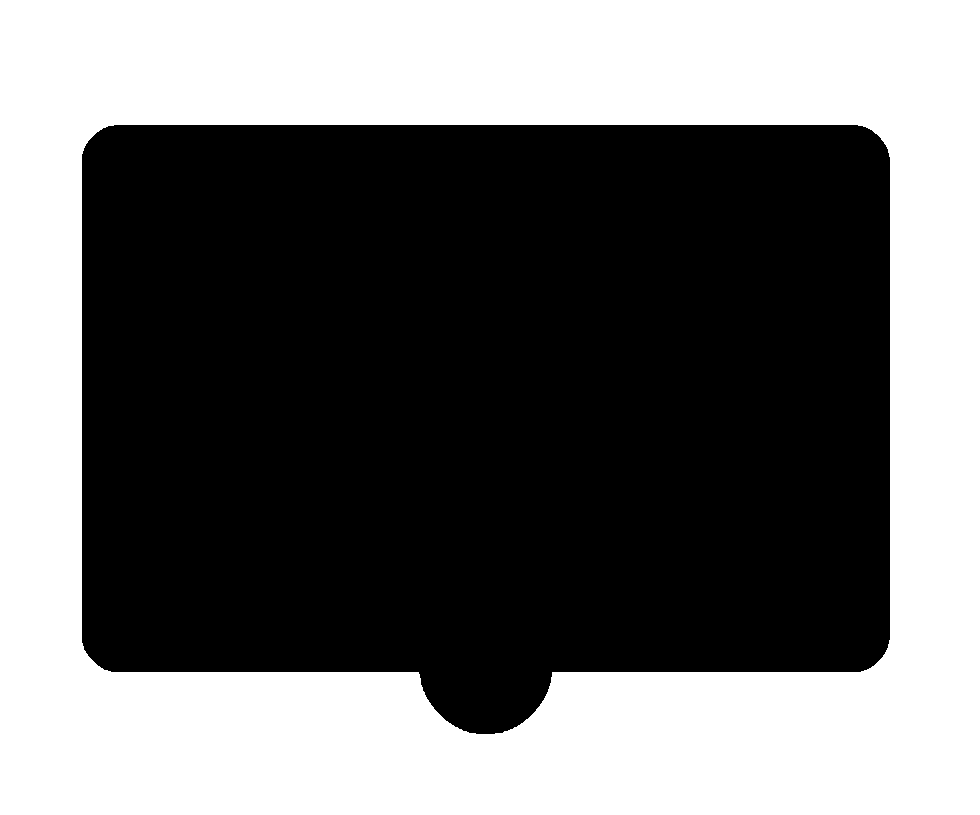
Add Values
Output Fields (0)
UNDERSTANDING THE SYSTEM
The behavior system uses Javascript as the primary programming language.
There are 6 snippets of code you will see in the editor, but the top 3 are the most important:
On Execute - This piece of code will run when the behavior is executed. It can be triggered by other behaviors or be triggered automatically on launch if no behavior is on the top (Root Behaviors).
On Disable - This piece of code will run when the behavior is disabled. The code will also be executed on launch when the behavior is initially disabled. Note that the "On Execute" script cannot be executed when the behavior is disabled - you may leave this blank if the behavior doesn't do anything when disabled.
On Enable - This piece of code will only execute when the behavior transitions from being disabled to being enabled via the Behavior On behavior. You may leave this blank if the behavior doesn't do anything when enabled - though, note that the behavior can trigger the "On Execute" script when enabled.
On Create - This piece of code will only execute once when the behavior has been created or cloned in a scene. It's the very first thing that runs once before anything happens. (eg. Box Container needs to yield an array before anything.)
On Destroy - This piece of code will only execute when the behavior's parent has been destroyed (not disabled) via Destroy Object. (eg. the Play Sound behavior still continues playing audio when destroyed but the audio is interrupted when disabled instead.)
Special - A special piece of code for the compiler to process, giving unique properties to behaviors. (eg. the Comment behavior can be executed when disabled.)
In the IDE, you can navigate through all scripts in a behavior via the Select Script dropdown at the top.
MANAGING THE BEHAVIOR
input[ inputField: STRING ]
The input variable is a dictionary containing the input fields of the behavior. The editor will show all existing input fields at the top of the page. Don't worry about data types or dynamic input fields - the system automatically converts them.
Example
yield( outputFields: DICTIONARY )
The yield function updates the output fields of the behavior using a dictionary where the keys are the output fields and the values are the values to update with. All output fields are shown at the bottom of the editor and must be all referenced in one call.
Example
trigger( force: BOOLEAN )
The trigger function executes all child behaviors. If force is enabled, the behaviors will be executed from left to right as used with Behavior Bundle. For behaviors like Loop and If, the function can be optional or be executed multiple times.
Example
internal[ key: STRING ]
The internal variable is a dictionary containing the behavior's internal data - this is a way that behaviors can "remember" their previous state and can act differently for each execution.
childrenCount
The childrenCount variable is a number representing the amount of child behaviors the behavior has.
triggerNode( node: NUMBER )
The triggerNode function executes a single child behavior. 0 will be the first child behavior. Exceeding the number of child behaviors will result in an error.
Example
behaviors[ name: STRING ][ function: STRING ]()
The behaviors variable is a dictionary where the key is the behaviorID and the value will contain functions. You can access other behaviors if necessary and perform actions on them.
All Usage Cases
global
The global variable is a boolean that is only true if the behavior is in the global world object.
root
The root variable is a boolean that is only true if the behavior is a root behavior. Root behaviors have no behaviors connected from the top, therefore the behavior executes on launch and can function differently.
Example
USING PHASER
game[ func: STRING ] ...
The Phaser 3 module will be heavily used throughout the entire project as the primary game engine. You can use it by referring to the game or PhaserScene variable. Check out Phaser's official documentation on how to use it!
Example